Adding custom fonts in React Native can elevate your app's design and user experience. In this blog post, we’ll walk you through adding custom fonts using Expo and then styling text using Tailwind CSS in your project.
Step 1: Download Font
First, head over to Google Fonts or any other font provider of your choice. Download the fonts you need in .ttf
format.
Example: Downloading Google Fonts
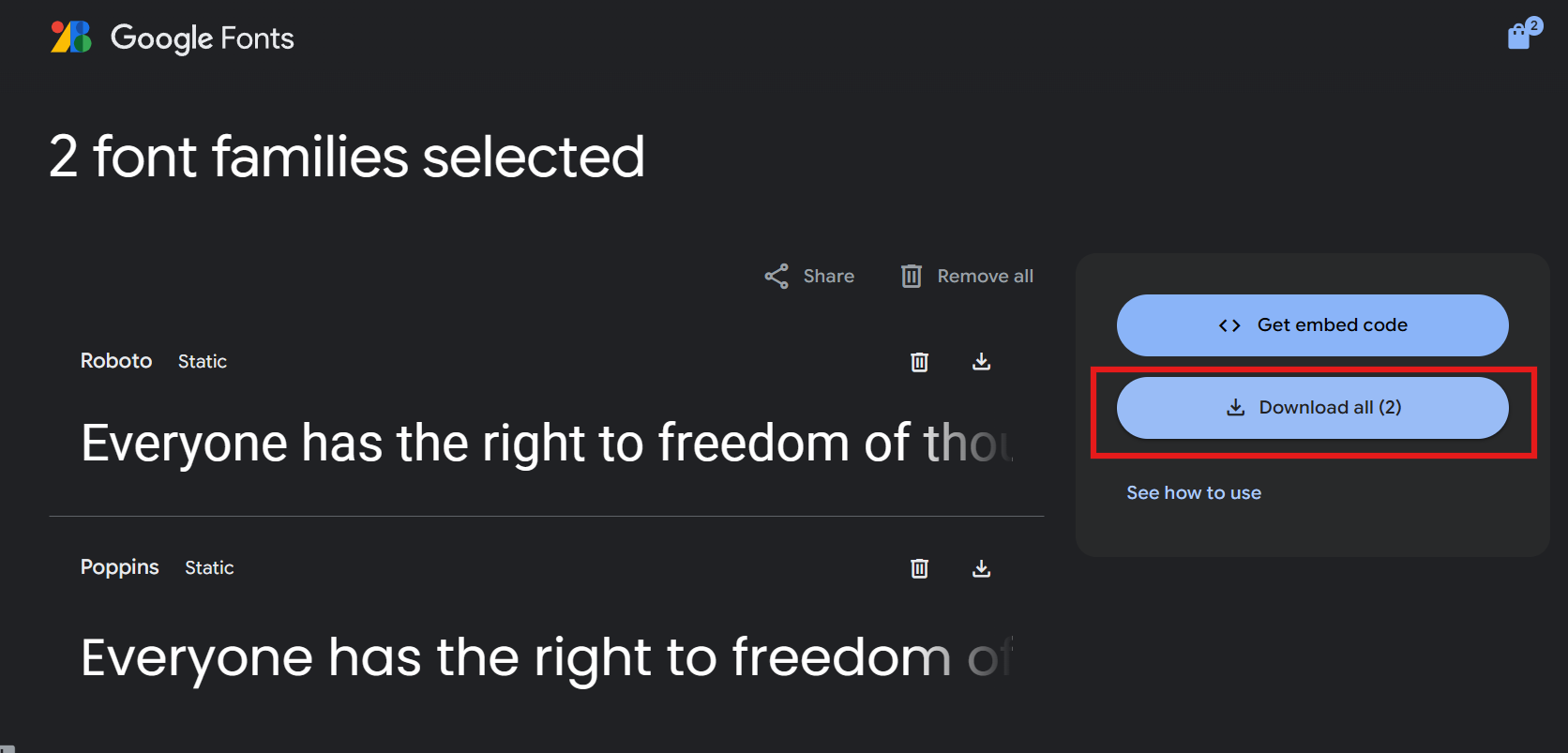
Step 2: Import Fonts into Your Project
To get started, place your downloaded font files in the assets/fonts
folder of your React Native project. If the assets
folder does not exist, create one in the root of your project directory.
YourProject/
├── assets/
│ └── fonts/
│ ├── SpaceMono-Regular.ttf
│ ├── ProductSans-Black.ttf
│ ├── ProductSans-Bold.ttf
│ ├── ProductSans-Light.ttf
│ ├── ProductSans-Medium.ttf
│ ├── ProductSans-Regular.ttf
│ ├── ProductSans-Thin.ttf
│ ├── ProductSans-Italic.ttf
Step 3: Configure Font Loading in RootLayout
Next, import the custom fonts using Expo's useFonts
hook in your layout component:
import { DarkTheme, DefaultTheme, ThemeProvider } from '@react-navigation/native';
import { useFonts } from 'expo-font';
import { Stack } from 'expo-router';
import * as SplashScreen from 'expo-splash-screen';
import { useEffect } from 'react';
import 'react-native-reanimated';
SplashScreen.preventAutoHideAsync();
export default function RootLayout() {
const colorScheme = useColorScheme();
// require fonts
const [loaded] = useFonts({
SpaceMono: require('../assets/fonts/SpaceMono-Regular.ttf'),
GBlack: require('../assets/fonts/ProductSans-Black.ttf'),
GBold: require('../assets/fonts/ProductSans-Bold.ttf'),
GLight: require('../assets/fonts/ProductSans-Light.ttf'),
GMedium: require('../assets/fonts/ProductSans-Medium.ttf'),
GRegular: require('../assets/fonts/ProductSans-Regular.ttf'),
GThin: require('../assets/fonts/ProductSans-Thin.ttf'),
GItalic: require('../assets/fonts/ProductSans-Italic.ttf'),
});
useEffect(() => {
if (loaded) {
SplashScreen.hideAsync();
}
}, [loaded]);
if (!loaded) {
return null;
}
return (
<ThemeProvider value={colorScheme === 'dark' ? DarkTheme : DefaultTheme}>
<Stack screenOptions={{ headerShown: false }}>
{/* Add your screens here */}
</Stack>
</ThemeProvider>
);
}
Explanation:
useFonts: Loads the custom fonts.
SplashScreen: Prevents the splash screen from hiding before fonts are loaded.
useEffect: Hides the splash screen once the fonts are loaded.
Implementation Example
import { View, Text } from 'react-native'
import React from 'react'
const index = () => {
return (
<View>
<Text style={{fontFamily: 'GRegular'}}>Loading</Text>
</View>
)
}
export default index
Step 4: Configure Tailwind Class for Custom Font
To use Tailwind CSS with custom fonts, you need to configure your tailwind.config.js
file.
/** @type {import('tailwindcss').Config} */
module.exports = {
content: ["./app/**/*.{js,jsx,ts,tsx}"], // Specify the paths to all of your components
presets: [require("nativewind/preset")],
theme: {
extend: {
// configure classname here
fontFamily: {
GBlack: ["GBlack"],
GBold: ["GBold"],
GLight: ["GLight"],
GMedium: ["GMedium"],
GRegular: ["GRegular"],
GThin: ["GThin"],
GItalic: ["GItalic"],
},
colors: {
graytext: "#374151",
secondary: "#E9EDF6",
},
},
},
plugins: [],
};
Explanation:
fontFamily: Extends the default theme to include your custom fonts.
colors: You can also add custom colors if needed.
Implementation Example
import { View, Text } from 'react-native';
import React from 'react';
const CustomFontExample = () => {
return (
<View className="!text-4xl text-center flex justify-center items-center">
<Text className="text-5xl text-red-400">Fonts</Text>
<Text className="font-GItalic text-4xl">Italic</Text>
<Text className="font-GBlack text-4xl">Black</Text>
<Text className="font-GBold text-4xl">Bold</Text>
<Text className="font-GLight text-4xl">Light</Text>
<Text className="font-GMedium text-4xl">Medium</Text>
<Text className="font-GRegular text-4xl">Regular</Text>
<Text className="font-GThin text-4xl">Thin</Text>
</View>
);
};
export default CustomFontExample;
Explanation:
Tailwind CSS Classes: We use Tailwind CSS classes (e.g.,
font-GItalic
,font-GBlack
) to apply different font styles.Text Styling: You can customize the text size, color, and font weight as needed.
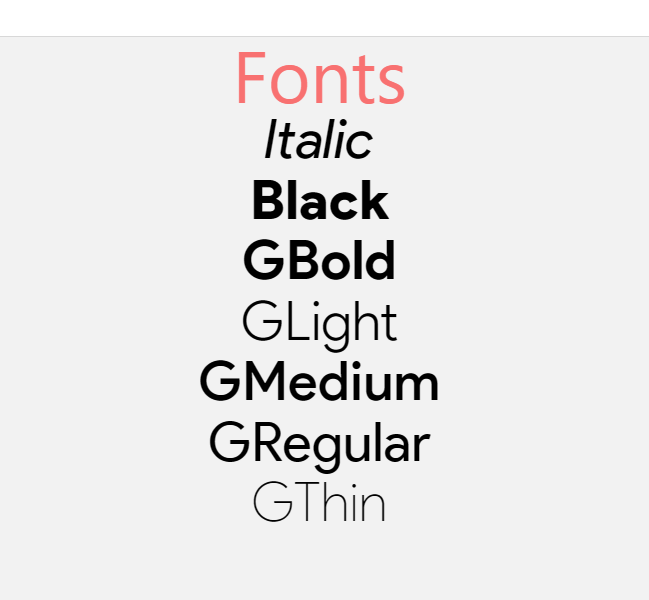
Final Thoughts
Customizing fonts in React Native can greatly enhance the user experience of your app. By following these steps and integrating Tailwind CSS, you have full control over your app’s typography. Experiment with different font styles to create a visually appealing UI.
Feel free to share your thoughts or ask questions in the comments below. Happy coding!