Tailwind CSS for React Native is known as NativeWind. it brings the power of Tailwind CSS to React Native, allowing you to use familiar Tailwind classes in your mobile applications. This guide will walk you through the setup process and show you how to start using Nativewind effectively.
Prerequisites
Before we begin, make sure you have:
A React Native project set up
Node.js installed
npm or yarn package manager
Installation Steps
1. Install Required Dependencies
First, install the necessary packages:
npx expo install nativewind tailwindcss react-native-reanimated react-native-safe-area-context
2. Create Tailwind Configuration
Run npx tailwindcss init
to create a tailwind.config.js
tailwind.config.js
/** @type {import('tailwindcss').Config} */
module.exports = {
// NOTE: Update this to include the paths to all of your component files.
content: ["./app/**/*.{js,jsx,ts,tsx}"],
presets: [require("nativewind/preset")],
theme: {
extend: {},
},
plugins: [],
}
3. Create Css File
Create a global.css
to root of project and add this code block.
global.css
@tailwind base;
@tailwind components;
@tailwind utilities;
4. Configure Babel
Update your babel.config.js
to include the Nativewind babel preset:
module.exports = function (api) {
api.cache(true);
return {
presets: [
["babel-preset-expo", { jsxImportSource: "nativewind" }],
"nativewind/babel",
],
};
};
5. Modify your metro.config.js
Generate the file by run this command npx expo customize
if it not exist.
const { getDefaultConfig } = require("expo/metro-config");
const { withNativeWind } = require('nativewind/metro');
const config = getDefaultConfig(__dirname)
module.exports = withNativeWind(config, { input: './global.css' })
6. Import your CSS file
before import Css file Remove the all unnecessary folders and files inside the app folder, only this two file is essential.
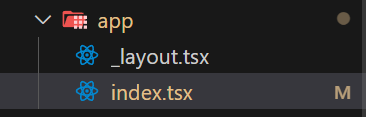
_layout.tsx
import React from 'react'
import { Stack } from 'expo-router'
import '../global.css' //import css file here
const RootLayout = () => {
return (
<Stack>
<Stack.Screen name='index' />
</Stack>
)
}
export default RootLayout
7. Set Up TypeScript (Optional)
If you're using TypeScript, create a file on Root with the name nativewind-env.d.ts
and this code.
nativewind-env.d.ts
/// <reference types="nativewind/types" />
8. implementation
index.tsx
import { View, Text } from 'react-native'
import React from 'react'
const index = () => {
return (
<View>
<Text className='bg-red-400 text-yellow-100'>hello it's me Nativewind</Text>
</View>
)
}
export default index
Folder Structure of project -
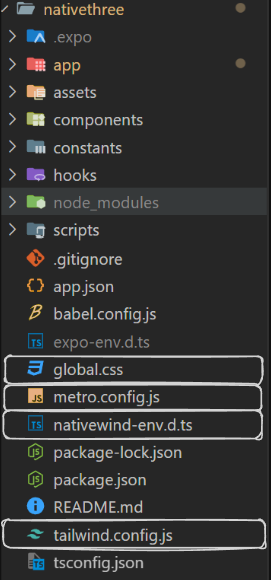
Troubleshooting
Common Issues and Solutions
Styles Not Applying
Verify babel configuration
Check content paths in tailwind.config.js
Ensure proper class names are being used
Build Errors
Clear metro bundler cache
Rebuild the project
Check for version compatibility
Conclusion
Nativewind brings the convenience of Tailwind CSS to React Native development. With proper setup and understanding of its capabilities, you can significantly speed up your mobile app development process while maintaining a consistent styling approach.
Remember to:
Keep your dependencies updated
Follow React Native best practices
Refer to the official documentation for advanced features
Happy coding!